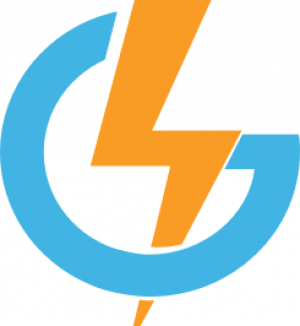
Here you can grab your free login manager for GameSparks in Unity that uses device’s id and random guid for auth.
As statistics say, you as gamedesigner shouldn’t use login or auth screens for player auth. Better auth them in background, using device unique Id’s or Google Play account (Play Games). That’s why I’ve written this script and published it for open use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
using UnityEngine; using UnityEngine.SceneManagement; using GameSparks.Api.Requests; using GameSparks.Api.Responses; using System; public class LoginManager : MonoBehaviour { public string SceneAfterLogin; public static LoginManager Instance; private void Awake() { Instance = this; } private void Start() { GameSparksManager.Instance.OnGameSparksInitializationCompleted.AddListener(TryAutoLogin); } private void TryAutoLogin() { if (PlayerPrefs.HasKey("login")) { Login(PlayerPrefs.GetString("login")); } } public static void Login(Action<string> registrationerror = null) { var login = new System.Guid().ToString(); Login(login, registrationerror); } public static void Login(string login, Action<string> registrationerror = null) { Login(login, SystemInfo.deviceUniqueIdentifier, Instance.Success, (s1, s2) => Register(s1, s2, Instance.Success, registrationerror)); } static void Login(string login, string password, Action success, Action<string, string> error = null) { new AuthenticationRequest() .SetUserName(login) .SetPassword(SystemInfo.deviceUniqueIdentifier) .Send((response) => { if (response.HasErrors) { if (error != null) { error(login, password); } } else { Debug.Log("Successful login"); PlayerPrefs.SetString("login", login); success(); } }); } static void Register(string login, string password, Action success, Action<string> error = null) { new RegistrationRequest() .SetUserName(login) .SetDisplayName(login) .SetPassword(SystemInfo.deviceUniqueIdentifier) .Send((response) => { if (response.HasErrors) { if (error != null) { error(login); } } else { Debug.Log("Successful registration"); success(); } }); } void Success() { SceneManager.LoadScene(SceneAfterLogin); } } |
GameSparks Manager that is used to fire event when GameSparks SDK is fully initialized.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
using GameSparks.Platforms; using UnityEngine; using UnityEngine.Events; public class GameSparksManager : MonoBehaviour { public static GameSparksManager Instance; public GameObject GameSparks; public UnityEvent OnGameSparksInitializationCompleted = new UnityEvent(); PlatformBase Platform; private void Awake() { if (Instance == null) { Instance = this; DontDestroyOnLoad(gameObject); } else { Destroy(gameObject); } } private void Update() { if (Platform != null) { return; } Platform = GameSparks.GetComponent<PlatformBase>(); if (Platform != null) { OnGameSparksInitializationCompleted.Invoke(); } } } |
369